Looping Through a List in JavaScript
Jul 16, 2022
Freddy
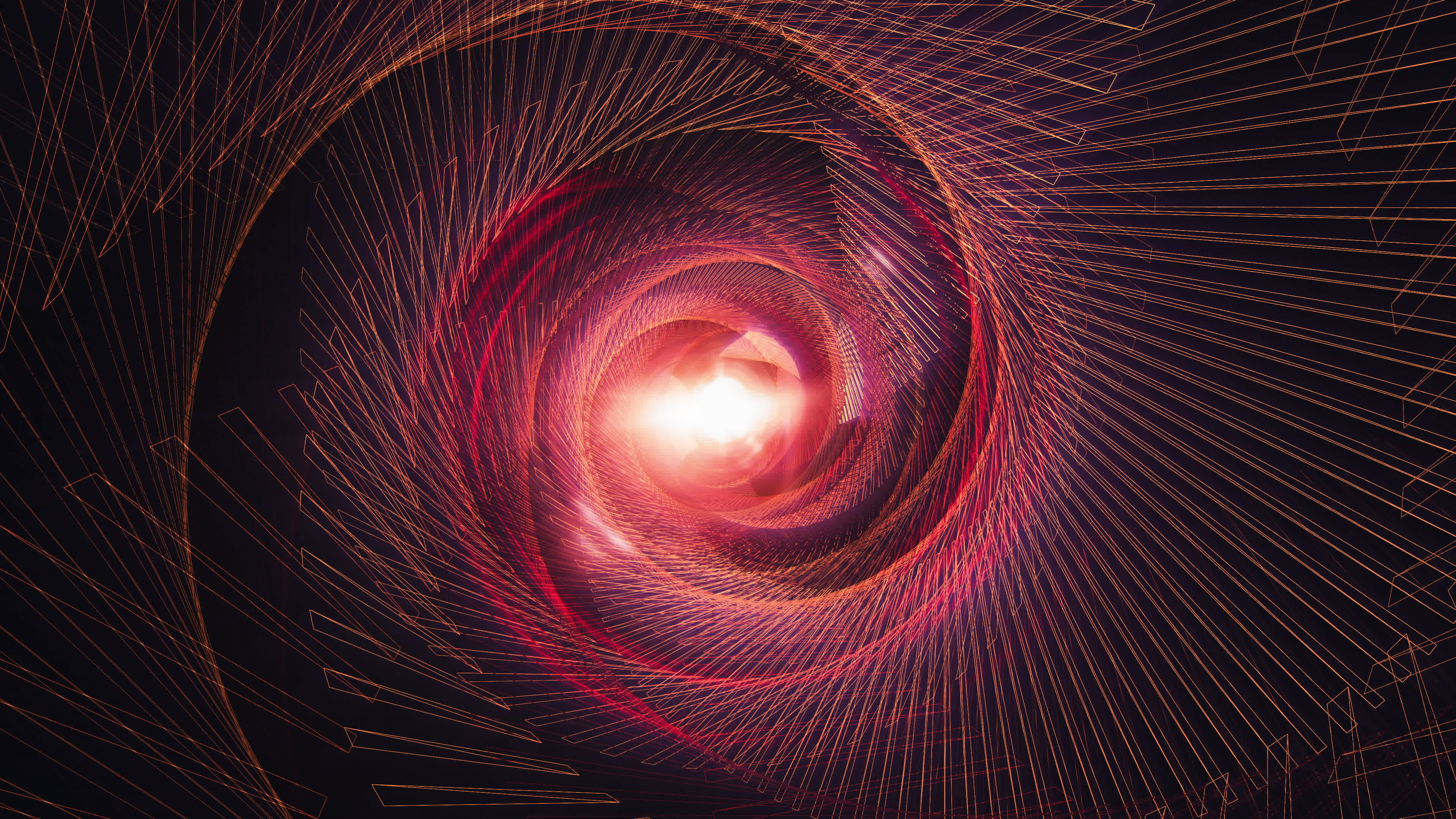
This short article aims to explore and compare the different ways of looping through a list in JavaScript: for, forEach and for of.
Say we have a list, and we want to loop through it.
const fruits = ["Apple", "Banana", "Cherry", "Date"];
Our aim is to print the list as such:
0. Apple
1. Banana
2. Cherry
3. Date
The familiar way
for (let i = 0; i < fruits.length; i++) {
console.log(i + ". " + fruits[i]);
}
This is the C-style for loop, as its syntax follows this pattern:
for (initialisation; condition; increment)
As a side note, JavaScript introduced template literals in 2015, allowing us to code more succinctly.
// Full code
const fruits = ["Apple", "Banana", "Cherry", "Date"];
for (let i = 0; i < fruits.length; i++) {
console.log(`${i}. ${fruits[i]}`);
}
forEach
The previous program is imperative: code is executed statement by statement.
JavaScript is (predominantly) an imperative language. However, the ES6 update in
2015 provided some functional tools, such as forEach
.
fruits.forEach(fruit => console.log(fruit));
What’s happening here? Well, JavaScript lists are objects, and a list object has
a forEach
method that takes in a function as a parameter. This function is
then invoked for each item in the list.
In fact, forEach
passes 2 parameters to the function: an item (compulsory) and
its index (optional). That said, we can achieve our aim with the following
program.
// Full code
const fruits = ["Apple", "Banana", "Cherry", "Date"];
fruits.forEach((fruit, i) => console.log(`${i}. ${fruit}`));
Unlike a for loop, forEach does not allow you to break out of the loop. forEach is also very slow. Many developers are against the usage of forEach - in any context.
for of
We move back to imperative programming with for of
, also introduced in ES6 in
2015.
for (let fruit of fruits) {
console.log(fruit);
}
Performance-wise, this is the fastest when it comes to small lists, but becomes very slow for large data sets. In this case, we also need the index, which makes the program slower.
// Full code
const fruits = ["Apple", "Banana", "Cherry", "Date"];
for (let [i, fruit] of fruits.entries()) {
console.log(`${i}. ${fruit}`);
}
Trivia
You may have noticed that I refer to fruits
as a list and not an array. This
is because fruits
is mutable: you can add, remove and modify the list. From a
theoretical point of view, arrays are immutable by definition. However, the
terms list and array have become synonymous within the JavaScript
community.
In fact, even the official documentation refers to them as arrays.
More Blog Posts
The Intuition behind Convolutional Neural Networks
The UCI Protocol for Chess Engines
Setting Up Unrestricted ChatGPT Locally on Mac
Histogram Equalisation for Colour Images
Enhancing Greyscale Image Contrast through Histogram Equalisation
On the Philosophy and Design Choices of this Site
Benchmarking Loops in JavaScript
Looping Through a List in JavaScript